Object Oriented Programming In PHP
Object-oriented programming is a programming style where group of functions or variables of a particular topic into a single unit. Object-oriented programming is considered to be more advanced and efficient than the procedural style of programming .
We can imagine our universe made of different objects like sun, moon, earth etc. Similarly we can imagine our car made of different objects like wheel, steering, engine etc. Same way there is object oriented programming concepts which assume everything as an object and implement a software using different objects .
Analysis – functionality of the system
Designing – architecture of the system
Programming – implementation of the application
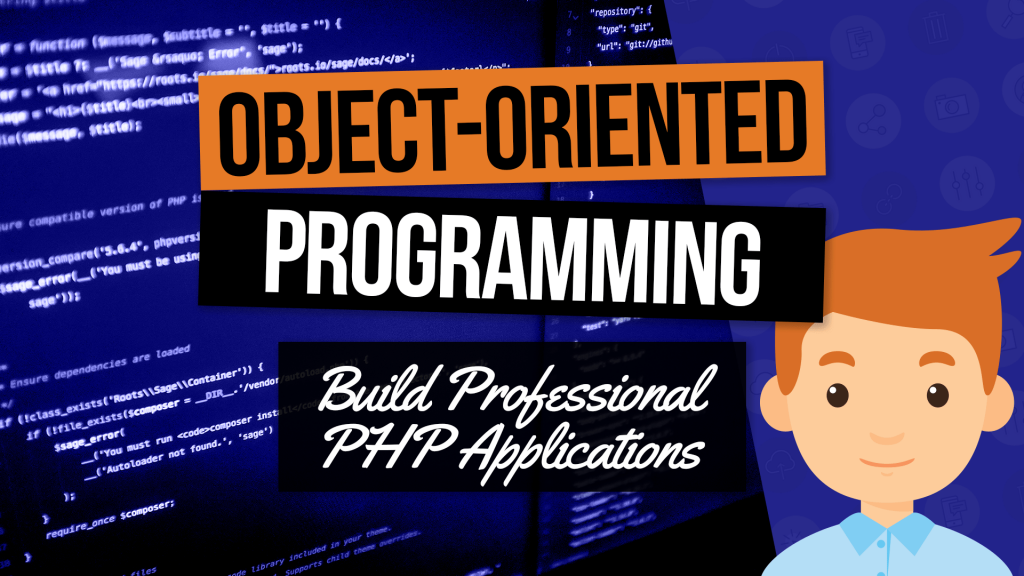
Here, we are going to take our first steps into the world of object oriented programming by learning the most basic terms in the field like classes, objects, properties, methods and much more.
Classes
A class holds the methods and properties shared by all of the objects that are created from class itself.
- First we have to define a php class, where classname should be same as filename.
- We declare the class with the class keyword and write the name of the class and capitalize the first letter.
- If the class name contains more than one word, we capitalize each word. This is known as upper camel case. For example, BankAccount, IndianIdol, SoftwareDeveloper etc.
- We circle the class body within curly braces. Inside the curly braces, we put our code.
Define PHP Classes
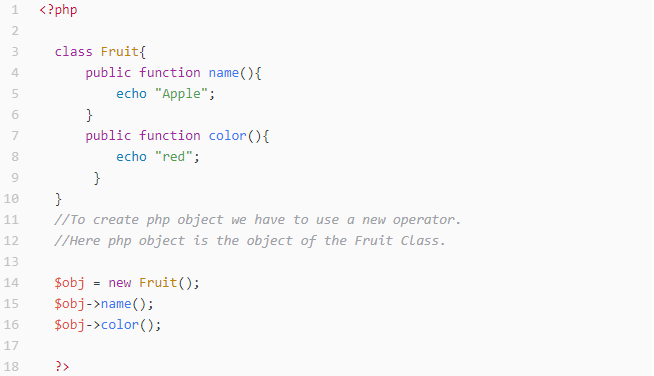
Here is the description of each line −
- The special form class, followed by the name of the class that you want to define.
- A set of braces enclosing any number of variable declarations and function definitions.
- Variable declarations start with the special form var, which is followed by a conventional $ variable name; they may also have an initial assignment to a constant value.
- Function definitions look much like standalone PHP functions but are local to the class and will be used to set and access object data.
Objects
- Object is an instance of a Class.
- You can instantiate an object, but not a Class. The process of creating an object is also known as instantiation.
- Although the objects share the same code, they can behave differently because they can have different values assigned to them.
- We can create several objects from the same class, with each object having its own set of properties.
In order to work with a class, we need to create an object from it. In order to create an object, we use the new keyword.
For example:

We created the object $bmw from the class Car with the new keyword.
We can create more than one object from the same class.

In fact, we can create as many objects as we like from the same class, and then give each object its own set of properties.
Properties (Member Variable )
These are the variables defined inside a class. This data will be invisible to the outside of the class and can be accessed via member functions. These variables are called attribute of the object once an object is created.
- We put the public keyword in front of a class property.
- The naming convention is to start the property name with a lower case letter.
- If the name contains more than one word, all of the words, except for the first word, start with an upper case letter. For example, $color or $isValid
- A property can have a default value.
For example,

We call properties to the variables inside a class. Properties can accept values like strings, integers, and booleans (true/false values), like any other variable.
Let’s add some properties to the Animal class.
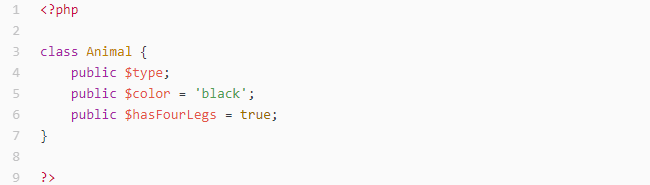
Methods (Member Functions)
These are the function defined inside a class and are used to access object data.
How to call member functions?
After creating your objects, you will be able to call member functions related to that object. One member function will be able to process member variable of related object only.
Following example shows how to set name and color for the two cars by calling member functions.
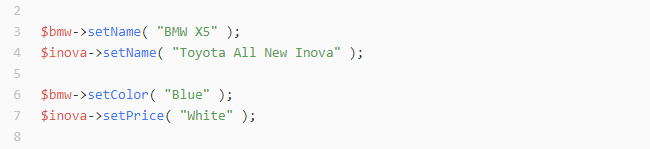
Now you call another member functions to get the values set by in above example :

This will produce the following result :

Constructor
PHP Constructor, If the class name and function name are similar, in that case function is known as constructor.Constructor Functions are special type of functions which are called automatically whenever an object is created. So we take full advantage of this behavior, by initializing many things through constructor functions.
PHP provides a special function called __construct() to define a constructor. You can pass as many as arguments you like into the constructor function.
The following example adds a constructor to the BankAccount class that initializes account number and an initial amount of money:
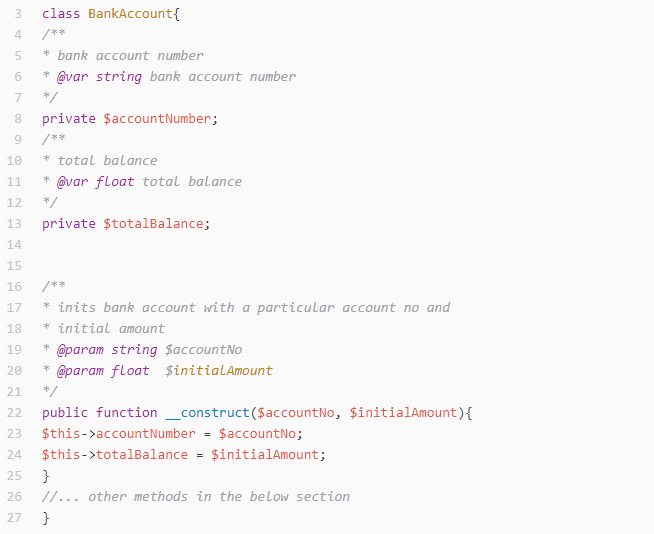
Now you can create a new bank account object with an account number and initial amount as follows:

PHP constructor overloading
Constructor overloading allows you to create multiple constructors with the same name __construct()
but different parameters. Constructor overloading enables you to initialize object’s properties in various ways. The following example demonstrates the idea of constructor overloading:
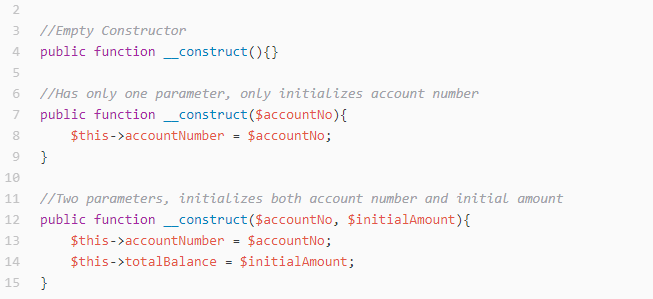
PHP have not yet supported constructor overloading in oops. Fortunately, you can achieve the same constructor overloading effect by using several PHP functions.
Let’s take a look at the following example:
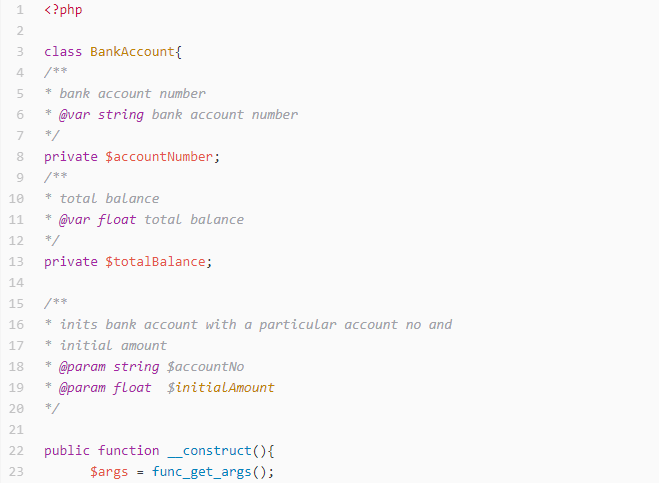

How the constructor works.
- First, we get constructor’s arguments using the func_get_args() function and also get the number of arguments using the func_num_args() function.
- Second, we check if the init_1() and init_2() method exists based on the number of constructor’s arguments using the method_exists() function. If the corresponding method exists, we call it with an array of arguments using the call_user_func_array() function.
Destructor
PHP destructor allows you to clean up resources before PHP releases the object from the memory. For example, you may create a file handle in the constructor and you close it in the destructor.
To add a destructor to a class, you just simply add a special method called __destruct() as follows:

There are some important notes regarding the destructor:
- Unlike a constructor, a destructor cannot accept any argument.
- Object’s destructor is called before the object is deleted. It happens when there is no reference to the object or when the execution of the script is stopped by the exit() function.
The following simple FileUtil class demonstrates how to use the destructor to close a file handle.
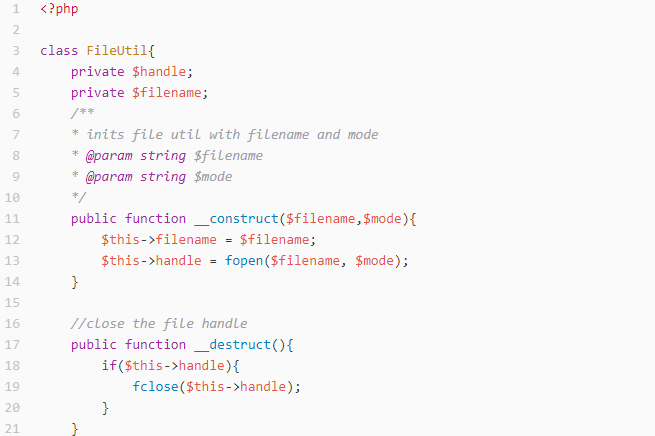
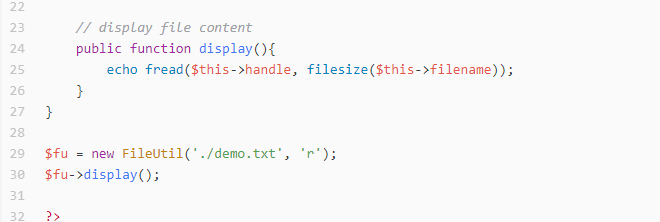
Constants
A constant is somewhat like a variable, in that it holds a value, but is really more like a function because a constant is immutable. Once you declare a constant, it does not change.
Declaring one constant is easy, as is done in below class :
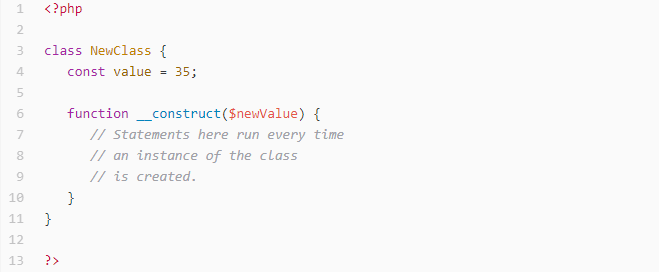
In this class, value is a constant. It is declared with the keyword const, and under no circumstances can it be changed to anything other than 35. Note that the constant’s name does not have a leading $, as variable names do.
Static Keyword
Declaring class members or methods as static makes them accessible without needing an instantiation of the class. A member declared as static can not be accessed with an instantiated class object (though a static method can).
Example :
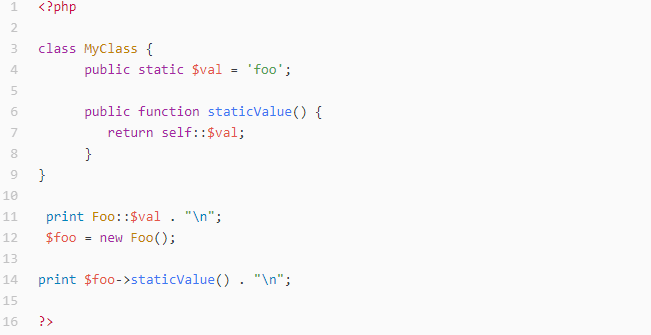
Final Keyword
PHP 5 introduces the final keyword, which prevents child classes from overriding a method by prefixing the definition with final. If the class itself is being defined final then it cannot be extended.
Example :
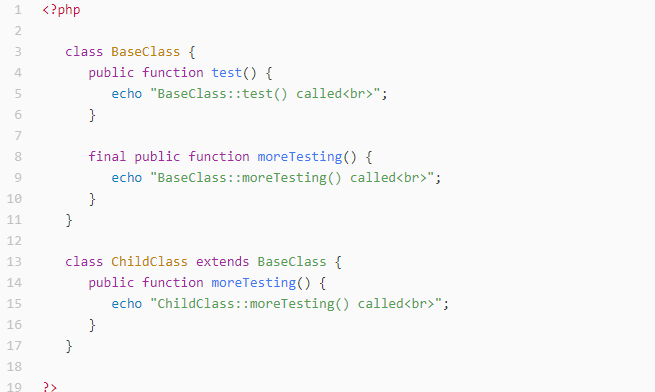
Result :
Fatal error: Cannot override final method BaseClass::moreTesting()
Why do I have to use final?
- Preventing massive inheritance chain of doom
- Encouraging composition
- Force the developer to think about user public API
- Force the developer to shrink an object’s public API
- A final class can always be made extensible
- extends breaks encapsulation
- You don’t need that flexibility
- You are free to change the code
When to avoid final:
Final classes only work effectively under following assumptions:
- There is an abstraction (interface) that the final class implements
- All of the public API of the final class is part of that interface
If one of these two pre-conditions is missing, then you will likely reach a point in time when you will make the class extensible, as your code is not truly relying on abstractions
Reasons :
- Declaring a class as final prevents it from being subclassed—period; it’s the end of the line.
- Declaring every method in a class as final allows the creation of subclasses, which have access to the parent class’s methods, but cannot override them. The subclasses can define additional methods of their own.
- The final keyword controls only the ability to override and should not be confused with the private visibility modifier. A private method cannot be accessed by any other class; a final one can.
Public Members
Unless you specify otherwise, properties and methods of a class are public. That is to say, they may be accessed in three possible situations :
- From outside the class in which it is declared
- From within the class in which it is declared
- From within another class that implements the class in which it is declared
Till now we have seen all members as public members. If you wish to limit the accessibility of the members of a class then you define class members as private or protected.
Private members
By designating a member private, you limit its accessibility to the class in which it is declared. The private member cannot be referred to from classes that inherit the class in which it is declared and cannot be accessed from outside the class.
A class member can be made private by using private keyword infront of the member.
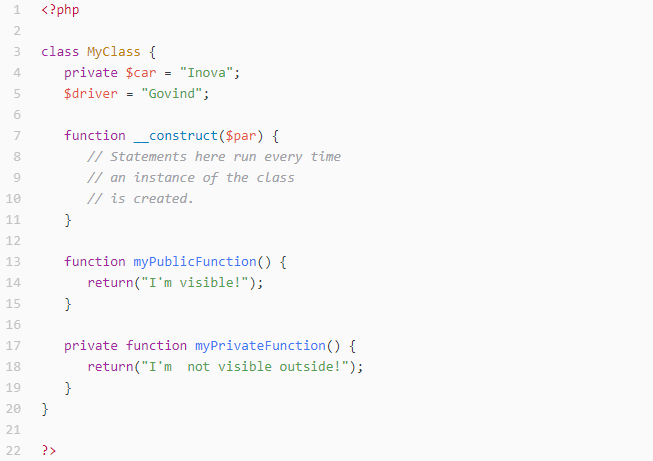
When MyClass class is inherited by another class using extends, myPublicFunction() will be visible, as will $driver. The extending class will not have any awareness of or access to myPrivateFunction and $car, because they are declared private.
Protected members
A protected property or method is accessible in the class in which it is declared, as well as in classes that extend that class. Protected members are not available outside of those two kinds of classes. A class member can be made protected by using protected keyword in front of the member.
Example :
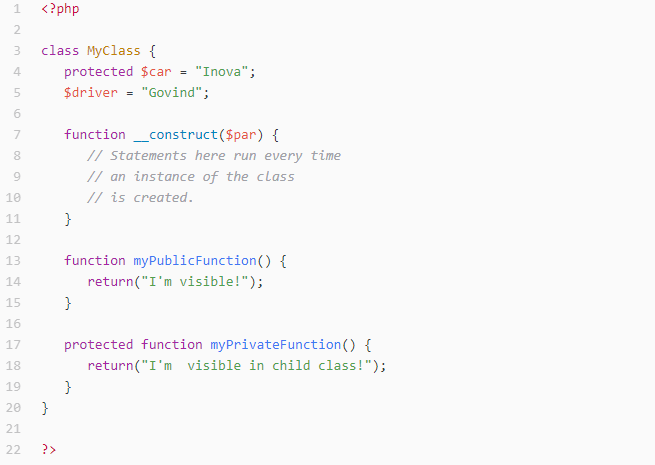
Object Oriented Programming Principles
The four major principles of OOP are Inheritance, Encapsulation, Polymorphism and Abstraction.
1. Inheritance
One of the main advantages of object-oriented programming is the ability to reduce code duplication with inheritance. Code duplication occurs when a programmer writes the same code more than once, a problem that inheritance strives to solve. In inheritance, we have a parent class with its own methods and properties, and a child class (or classes) that can use the code from the parent. By using inheritance, we can create a reusable piece of code that we write only once in the parent class, and use again as much as we need in the child classes.
Inheritance allows us to write the code only once in the parent, and then use the code in both the parent and the child classes.
In order to declare that one class inherits the code from another class, we use the extends keyword.
Lets, take an below example :
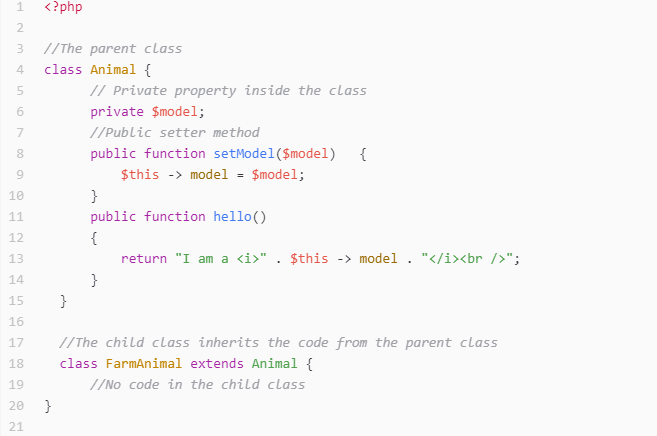
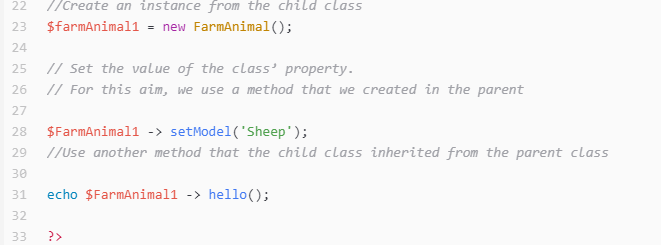
Result:
I am a Sheep
In the example given below, the FarmAnimal class inherits the Animal class, so it has access to all of the Animal’s methods and properties that are not private. This allows us to write the setModel() and hello() public methods only once in the parent, and then use these methods in both the parent and the child classes.
The result reflects the fact that the hello() method from the parent class was overridden by the child method with the same name.
2. Encapsulation
This is concerned with hiding the implementation details and only exposing the methods. The main purpose of encapsulation is to :
- Reduce software development complexity
By hiding the implementation details and only exposing the operations, using a class becomes easy. - Protect the internal state of an object
Access to the class variables via “get” and “set” methods, this makes the class flexible and easy to maintain.
The internal implementation of the class can be changed without worrying about breaking the code that uses the class.
Lets take an Example of Animal Class :
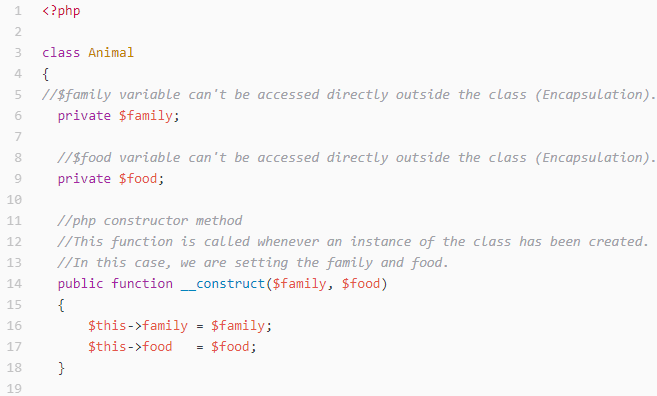
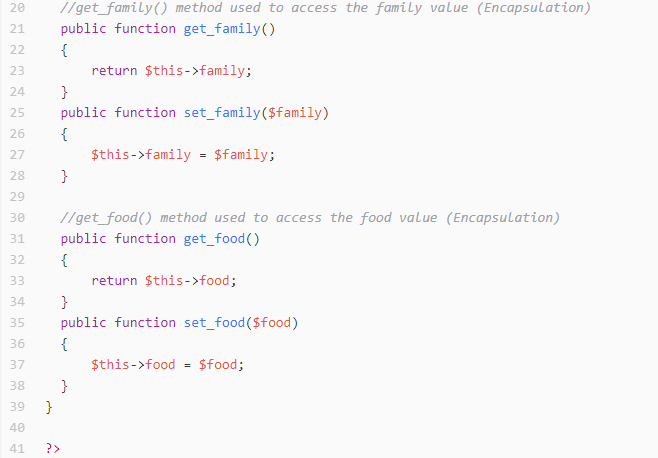
3. Polymorphism
This is an object oriented concept where same function can be used for different purposes. For example function name will remain same but it take different number of arguments and can do different task.
- Overloading
Same method name with different signature, since PHP doesn’t support method overloading concept - Overriding
When same methods defined in parents and child class with same signature i.e know as method overriding
The beauty of polymorphism is that the code working with the different classes does not need to know which class it is using since they’re all used the same way.
In the programming world, polymorphism is used to make applications more modular and extensible. Instead of messy conditional statements describing different courses of action, you create interchangeable objects that you select based on your needs. That is the basic goal of polymorphism.
For example, we can call the method that calculates the area calcArea() and decide that we put, in each class that represents a shape, a method with this name that calculates the area according to the shape. Now, whenever we would want to calculate the area for the different shapes, we would call a method with the name of calcArea() without having to pay too much attention to the technicalities of how to actually calculate the area for the different shapes. The only thing that we would need to know is the name of the method that calculates the area.
Implement the polymorphism principle
In order to ensure that the classes do implement the polymorphism principle, we can choose between one of the two options of either abstract classes or interfaces.
In the example given below, the interface with the name of Shape commits all the classes that implement it to define an abstract method with the name of calcArea().
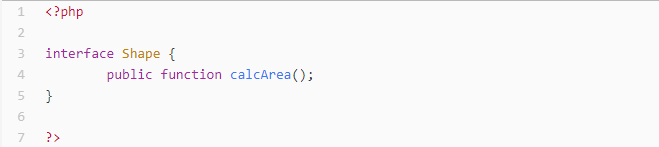
In accordance, the Circle class implements the interface by putting into the calcArea() method the formula that calculates the area of circles.
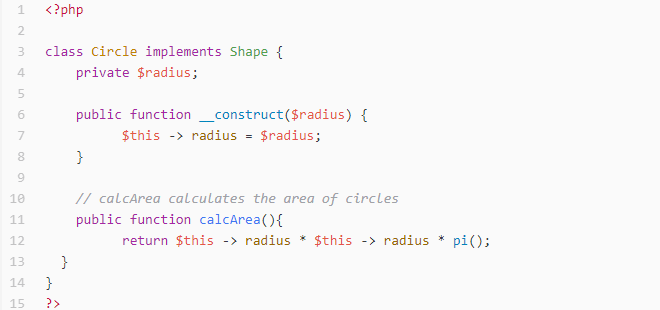
The rectangle class also implements the Shape interface but defines the method calcArea() with a calculation formula that is suitable for rectangles:
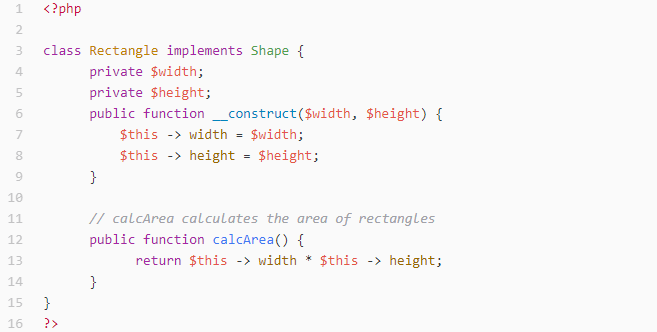
Now, we can create objects from the concrete classes:
$circ = new Circle(3); $rect = new Rectangle(3,4);
We can be sure that all of the objects calculate the area with the method that has the name of calcArea(), whether it is a rectangle object or a circle object (or any other shape), as long as they implement the Shape interface.
Now, we can use the calcArea() methods to calculate the area of the shapes:
echo $circ -> calcArea();
echo $rect -> calcArea();
Result:
28.274333882308
12